File Operations in Visual Basic

A common issue in Visual Basic is how to perform file operations, for instance, how to copy or delete a file, rename it or create a directory or folder. This page discusses them, and also the errors that may occur. The image on the right shows the Code VBA add-in menu for managing files. The software can be downloaded here.
Copying Files
When you copy a file you keep the existing file, and make a copy of the file with a new name. Visual Basic provides a statement for this called FileCopy.
where source is the name of the file to copy, and destination is the name of the copied file.
You have several choices when it comes to specifying the file names here, you can use the full path names for the files, or you can just specify the name of the files.
By way of background, Windows keeps track of something called the current drive and the current directory for us , these are basically pointers in the File System, and in the old days of DOS allowed us to perform mundane file operations without having to specify the name of the Drive and the Directory. These pointers still carry on in VB and Windows, so if we use this syntax in the Immediate window
FileCopy "a.txt", "b.txt"
Windows looks for a file called "a.txt" in the current drive and current directory of our PC, and if the operating system finds it, copies the file as "b.txt", again in the default drive and directory of the PC.
The problem here is that if the file is not found, your program bombs, just like this…

The Current Drive and Current Directory
As it turns out, Visual Basic has a function that can be used to determine the current directory called the CurDir function.
Debug.Print "The current directory is " & CurDir
returns C:\Users\peters\Documents
Changing the Current Drive and Current Directory
Once you know the current directory, you can then use the ChDir and ChDrive functions to change either the current drive or the current directory, like this…
ChDrive ("d")
ChDir "\vbfiles"
Debug.Print "The current directory is " & CurDir
Now if you are like me, you may not want to leave anything to chance, in which case, you can use the full path name with the FileCopy statement, like this…
FileCopy "c:\vbfiles\a.txt", "c:\vbfiles\b.txt"

If you attempt to copy a file that is opened, you'll receive run-time error '70': Permission denied
Does a file exist?
There's no confirmation that the copy was successful, but you can determine if a file exists by using the Visual Basic Dir$ function. The Dir$ function requires just a single argument representing the file name (as was the case with the CopyFile statement, you can specify just the file name or the full path name). If the file is found, then Dir$ returns the file name (not the full path). If the file is not found, then Dir$ returns an empty string. Let's see how we can use the Dir$ function to determine if a file exists before we copy it.
Dim retval As String
retval = Dir$("c:\vbfiles\b.txt")
If retval = "b.txt" Then
MsgBox "b.txt exists - no need to copy it..."
Else
FileCopy "c:\vbfiles\a.txt", "c:\vbfiles\b.txt"
End If
We receive a message saying that the file already exists, Dir$ has done its job.
By the way, you'll discover that there's a Dir function as well, Dir returns a variant return value and Dir$ returns a string.
Renaming Files
Renaming files is similar to copying them--this time we use the Visual Basic Name statement. As was the case when we copied files, we can choose either to specify a file name or to include the full path name, once again, I advise the full path name…
Name "c:\vbfiles\b.txt" As "c:\vbfiles\newb.txt"
This code will result in the file 'b.txt' begin renamed to 'newb.txt'. Once again, don't expect a confirmation message telling you that the rename was successful--the only message you'll receive is if the file does not exist

Deleting Files
The final file operation I'll discuss in this article is that of deleting a file. Visual Basic provides us with the Kill statement which will delete a file (and dangerously, a wildcard selection of files) of our choosing. Let's say that we wish to delete the file ''newb.txt' file that we created just a few minutes ago. This code will do the trick…
Kill "c:\vbfiles\newb.txt"
Again, there will be no confirmation message, only an error message if the file we are attempting to delete does not exist.
As I mentioned, you can also use wildcards as an argument to the Kill statement (WARNING: Don’t attempt this at home!!!). For instance, this code will delete EVERY file in the \VBFILES directory that has a file extension of *.txt…
Kill "c:\vbfiles\*.txt"
Most dangerously, this code will delete EVERY file in the \VBFILES directory…
Kill "c:\vbfiles\*.*"
Be careful when using the Kill statement, when issued through Visual Basic, there's no going back. There is no Undo statement, and files deleted in this way are NOT moved to the Windows Recycle bin.
Moving Files
There is no explicit Visual Basic statement to move a file. To simulate a move of a file, all we need to do is combine the FileCopy and Kill statements that we've already seen. For instance, to move the file a.txt from C:\VBFILES to C:\VBILES\CHINA, we can execute this code…
FileCopy "c:\vbfiles\a.txt", "c:\vbfiles\china\a.txt"
Kill "c:\vbfiles\a.txt"
Again, don't expect any confirmation messages, only errors if the files you reference do not exist.
That's it for Visual Basic actions that we can take against files, now it's time to turn our attention to Directory or folder operations.
Creating a Directory (Folder)
Creating a Folder is something that we're used to doing using Windows Explorer, but Visual Basic gives us the capability of creating folders within our program using the MkDir statement.
where path is either the name of a folder to be created, or (better yet!) the full path name of the directory or folder that you wish to create.
Specifying just the folder name to be created can be dangerous, if you are not aware of the current drive and directory, you may wind up creating a folder somewhere on your hard drive, with no real idea where it went. Better to be sure and specify the full path name, like this
MkDir "c:\vbfiles\code"
This code will create a folder called 'code' within the folder 'vbfiles' on the C Drive. Once again, you'll receive no confirmation message if the folder is created, but you will receive an error message if the folder creation fails.
There are two potential errors when executing the MkDir statement.
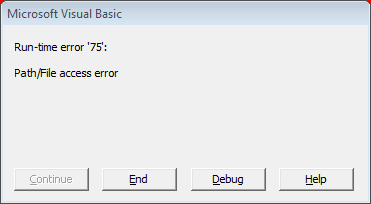
First, if you attempt to create a folder that already exists, you'll receive error 75 path file access error.
The error message is not explicit enough for my liking, but that's what it means, the folder 'code' already exists.
A second possible pitfall is attempting to create a folder within a folder that itself does not exist. For instance, in this code
MkDir "c:\vbfiles\code\one\two"
if the folder 'one' does not yet exist within 'code', you can't create the folder 'two' and you'll receive error message 76 path not found
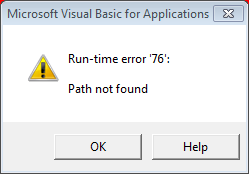
Removing a Directory (Folder)
Removing a directory is similar to removing a file, in this case, we use the Visual Basic RmDir statement. As was the case with the MkDir statement, path can either be a file name or the full path of a file name (once again, my recommendation). This code will remove the folder 'code' that we just created …
RmDir "c:\vbfiles\code"
It should come as no surprise to you that there's no confirmation message generated for a successful removal of the folder.
Possible error messages from RmDir?
There are two pitfalls. First, as we've seen all along, if you attempt to remove a folder that does not exist, you'll receive this error message 76 path not found
A second possible error can occur if you attempt to remove a directory or folder that contains files. If you try, you'll receive error 75 path file access error.
You must first use the Kill statement to remove every file from the folder before executing the RmDir statement (that's where the wildcard for the Kill statement comes in handy!)
Moving a Directory (Folder)
As was the case with moving files, there is no explicit Visual Basic statement that will do this for you.. To move a folder (and everything along with it), you'll first need to create the new folder, then use FileCopy to copy all of its files to the new folder, then delete all the files in the old folder using the Kill statement, and finally remove the old folder.