Getting username, special folders and more
using the Environ function
The Environ function lets you get the Windows environment variables for the computer your program is currently running on, for example the user name or the name of the temporary folder. Below you will find a summary of the system variables available, their meaning - if not self-evident and an example af what value to expect when calling the Environ function for that variable. The summary is organized in three groups:
A common use is to get the "ComputerName" value to see from which computer the user is logging on, MsgBox Environ("ComputerName")
. The screenshots show how you can insert the code easily using the Code VBA add-in. At the bottom of this page you will find VBA code to determine which system variables are available on your PC.
Warning |
---|
System Environment Variables may be edited by your windows user, which, in some cases, may compromise their integrity. For cases where the developer absolutely must have the actual system value, regardless of what changes may have been made to the environment variables by the user of the computer, it is recommended to use the APIs provided for this information. |
Using Environ to get special folders
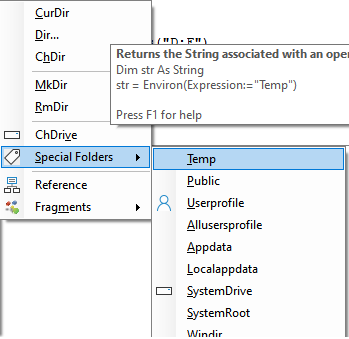
- Temporary folder
- Public folder
- User's profile folder
- All Users" or "Common" profile folder
- AppData\Roaming folder
- AppData\Local folder
- home drive and path
- Program Files folder
Using Environ to get the Temporary folder
The default temporary folder that is used by the operating system and applications available to the currently logged on user
Environ("TEMP")
(or Environ("TMP")
- which should be the same)
example result: C:\Users\johndoe\AppData\Local\Temp
Using Environ to get the Public Folder
The Public Folder is a Windows folder that you can use to share files with other people that either use the same computer, or connect to it over a network.Environ("PUBLIC")
example result: C:\Users\Public)
Using Environ to get the user's profile folder
Environ("USERPROFILE")
example result: C:\Users\johndoe
Using Environ to get the "All Users" or "Common" profile folder
The AllUsersProfile environment variable points to location of the "All Users" or "Common" profile folder. Paths are localized.Environ("ALLUSERSPROFILE")
example result: C:\ProgramData
Using Environ to get the AppData\Roaming folder
AppData environment variable points to the base location of where applications should store their data by default. It is a subdirectory of the user's profile folder.Environ("APPDATA")
example result: C:\Users\johndoe\AppData\Roaming
Using Environ to get LOCALAPPDATA
LocalAppData environment variable refers to location of temporary files of applications. Its uses include storing of desktop themes, Windows error reporting, caching and profiles of web browsers.Environ("LOCALAPPDATA")
example result: C:\Users\johndoe\AppData\Local
Using Environ to get SystemDrive
SystemDrive environment variable is a special system-wide environment variable, its value is the drive upon which the system folder was placed.Environ("SystemDrive")
example result: C:
Using Environ to get SystemRoot
The location of the system folder, including the drive and path.Environ("SystemRoot")
example result: C:\Windows
Using Environ to get HOMEDRIVE
HomeDrive environment variable returns drive letter on the local computer that is connected to the user's home directory. The drive letter to use is defined in the user's account properties within the domain. This environment variable is based on the value of the home directory. If the home directory is UNC, this variable will be populated with the drive letter that should be mapped to the UNC. If the home directory is a local path, this variable will be populated with the drive letter of the local pathEnviron("HOMEDRIVE")
example result: C:
Using Environ to get HOMEPATH
HomePath environment variable returns the complete path of the user's home directory, as defined in the user's account properties within the domain. HomePath environment variable is based on the value of the home directory. If the home directory is a UNC, this variable will will return the path (example: "\mypath") to the user's home directory using the mapped drives as a root. IF the home directory uses a local path, it will return the drive letter of that local path.Environ("HOMEPATH")
example result: \Users\johndoe
Using Environ to get the Windows directory
Environ("windir")
example result:C:\Windows
Using Environ to get ProgramFiles
ProgramFiles environment variable points to Program Files directory, which stores all the installed program of Windows and others. THe value of the ProgramFiles variable depends on whether the process requesting the environment variable is itself 32-bit or 64-bit (this is caused by Windows-on-Windows 64-bit redirection)Environ("ProgramFiles")
example result: C:\Program Files (x86))
Note |
---|
The default value of ProgramFiles environment variable on English-language systems is C:\Program Files. In 64-bit editions of Windows there are also ProgramFiles(x86) environment variable which defaults to C:\Program Files (x86) and ProgramW6432 environment variable which defaults to C:\Program Files. |
Using Environ to get CommonProgramFiles
Environ("CommonProgramFiles")
example result: C:\Program Files (x86)\Common Files")
Using Environ to get user info
Using Environ to get user name / logon ID
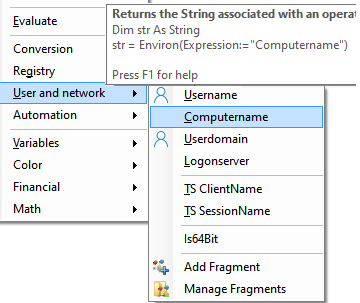
Environ("USERNAME ")
example result: johndoe
Using Environ to get the computer name
The NetBOIS name of the computer.Environ("COMPUTERNAME")
example result:BETA
Using Environ to get USERDOMAIN
UserDomain environment variable points to the name of the domain that contains the user's account.Environ("USERDOMAIN")
example result: Intranet
Using Environ to get the Terminal Services ClientName
Returns value of ComputerName environment variable of a remote host. The ClientName environment variable is Terminal Services specific, and only appears in a user's environment when they are logged on to a Terminal server, in Remote Administration or Application Server mode.Environ("ClientName")
example result:MyServer
Using Environ to get Terminal Services SessionName
The SessionName variable is only defined if the Terminal Services system component is installed to the Windows XP clients or newer. [1] When a client connects via a terminal server session, SessionName environment variable is a combination of the connection name, followed by a pound symbol {#} and then the session number.Environ("SESSIONNAME")
example result: Console
Using Environ to get LOGONSERVER
LogonServer environment variable indicates which domain controller authenticated the client's logon request.Environ("LOGONSERVER")
example result: \\BETA
Using Environ to get the computer's OS and processor info
Using Environ to get OS
OS environment variable returns the operating system name. Windows 2000 and later versions of Microsoft Windows display the operating system as Windows_NT.Environ("OS")
example result: Windows_NT
Using Environ to get PROCESSOR_ARCHITECTURE
Processor_Architecture environment variable indicates the chip architecture of the CPU. Values: x86, IA64.[Environ("PROCESSOR_ARCHITECTURE")
example result: x86:
Using Environ to get PROCESSOR_ARCHITEW6432
Environ("PROCESSOR_ARCHITEW6432")
example result: AMD64)
Using Environ to get PROCESSOR_IDENTIFIER
Processor_Identifier environment variable returns a description of the CPU, for example: "x86 Family 15 Model 2 Stepping 9, GenuineIntel".[Environ("PROCESSOR_IDENTIFIER")
example result: Intel64 Family 6 Model 30 Stepping 5, GenuineIntel
Using Environ to get PROCESSOR_LEVEL
Processor_Level environment variable indicates the model number of the CPU installed in the computer, including x86 = 3,4,5 and higher; Alpha = 21064; and MIPS = 3000 or 4000.Environ("PROCESSOR_LEVEL")
example result: 6
Using Environ to get PROCESSOR_REVISION
Processor_Revision environment variable returns the revision number of the CPU installed in the computer.Environ("PROCESSOR_REVISION")
example result: 1e05
Using Environ to get NUMBER_OF_PROCESSORS
Number_Of_Processors environment variable specifies the number of processors installed on the computer.Environ("NUMBER_OF_PROCESSORS")
example result: 8
Code to determine which system variables are available on your PC / in your profile
Sub ListEnvironVariables()
Dim strEnviron As String
Dim i As Long
For i = 1 To 255
strEnviron = Environ(i)
If LenB(strEnviron) = 0& Then Exit For
Debug.Print strEnviron
Next
End Sub
NB If you get the error Library or property not found, use VBA.Environ() or VBA.Environ$() to avoid it.
Running the above code on my PC returned:
ALLUSERSPROFILE=C:\ProgramData APPDATA=C:\Users\uildriks\AppData\Roaming asl.log=Destination=file CLASSPATH=.;C:\Program Files (x86)\Java\jre6\lib\ext\QTJava.zip CommonProgramFiles=C:\Program Files (x86)\Common Files CommonProgramFiles(x86)=C:\Program Files (x86)\Common Files CommonProgramW6432=C:\Program Files\Common Files COMPUTERNAME=BETA ComSpec=C:\Windows\system32\cmd.exe CSSCRIPT_DIR=C:\projects\ThirdParty\cs-script CSSCRIPT_SHELLEX_DIR=C:\ProgramData\CS-Script\ShellExtension\3.3.0.0\CS-Script DXROOT=C:\Program Files (x86)\Sandcastle\ EMC_AUTOPLAY=c:\Program Files (x86)\Common Files\Roxio Shared\ FP_NO_HOST_CHECK=NO HOMEDRIVE=C: HOMEPATH=\Users\uildriks LOCALAPPDATA=C:\Users\uildriks\AppData\Local LOGONSERVER=\\BETA NUMBER_OF_PROCESSORS=8 OS=Windows_NT Path=C:\Program Files (x86)\Microsoft Office\Office14\;C:\projects\ThirdParty\cs-script\lib;C:\projects\ThirdParty\cs-script;C:\Program Files\Common Files\Microsoft Shared\Windows Live;C:\Program Files (x86)\Common Files\Microsoft Shared\Windows Live;C:\Windows\system32;C:\Windows;C:\Windows\System32\Wbem;C:\Windows\System32\WindowsPowerShell\v1.0\;c:\Program Files (x86)\Common Files\Roxio Shared\10.0\DLLShared\;c:\Program Files (x86)\Common Files\Roxio Shared\DLLShared\;C:\Program Files\Microsoft Windows Performance Toolkit\;C:\Program Files (x86)\Windows Live\Shared;c:\Program Files (x86)\Microsoft ASP.NET\ASP.NET Web Pages\v1.0\;c:\Program Files (x86)\Microsoft SQL Server\100\Tools\Binn\;c:\Program Files\Microsoft SQL Server\100\Tools\Binn\;c:\Program Files\Microsoft SQL Server\100\DTS\Binn\;c:\Program Files (x86)\Microsoft SQL Server\100\Tools\Binn\VSShell\Common7\IDE\;c:\Program Files (x86)\Microsoft SQL Server\100\DTS\Binn\;C:\Program Files (x86)\QuickTime\QTSystem\;C:\Program Files\Microsoft SQL Serve r\110\Tools\Binn\;C:\Program Files\Microsoft\Web Platform Installer\;C:\Program Files (x86)\Microsoft Visual Studio 2008\SDK\VisualStudioIntegration\Tools\Sandcastle\ProductionTools\;C:\Program Files (x86)\Microsoft Visual Studio 2008 SDK\VisualStudioIntegration\Tools\Sandcastle\ProductionTools\ PATHEXT=.COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH;.MSC PROCESSOR_ARCHITECTURE=x86 PROCESSOR_ARCHITEW6432=AMD64 PROCESSOR_IDENTIFIER=Intel64 Family 6 Model 30 Stepping 5, GenuineIntel PROCESSOR_LEVEL=6 PROCESSOR_REVISION=1e05 ProgramData=C:\ProgramData ProgramFiles=C:\Program Files (x86) ProgramFiles(x86)=C:\Program Files (x86) ProgramW6432=C:\Program Files PSModulePath=C:\Windows\system32\WindowsPowerShell\v1.0\Modules\ PUBLIC=C:\Users\Public QTJAVA=C:\Program Files (x86)\Java\jre6\lib\ext\QTJava.zip RoxioCentral=c:\Program Files (x86)\Common Files\Roxio Shared\10.0\Roxio Central36\ SESSIONNAME=Console SystemDrive=C: SystemRoot=C:\Windows TEMP=C:\Users\uildriks\AppData\Local\Temp TMP=C:\Users\uildriks\AppData\Local\Temp USERDOMAIN=beta USERNAME=uildriks USERPROFILE=C:\Users\uildriks VS100COMNTOOLS=c:\Program Files (x86)\Microsoft Visual Studio 10.0\Common7\Tools\ VS90COMNTOOLS=c:\Program Files (x86)\Microsoft Visual Studio 9.0\Common7\Tools\ VSSDK100Install=C:\Program Files (x86)\Microsoft Visual Studio 2010 SDK\ VSSDK90Install=C:\Program Files (x86)\Microsoft Visual Studio 2008 SDK\ WecVersionForRosebud.188C=4 windir=C:\Windows WIX=C:\Program Files (x86)\WiX Toolset v3.6\